Assignment 8: Word search
Due Friday, November 20, before midnight Extended! Due November 22, before midnight
The goals for this assignment are:
-
Define and use functions and objects
-
Use binary and linear search
1. Description
The goal of this assignment is to create a word search game.
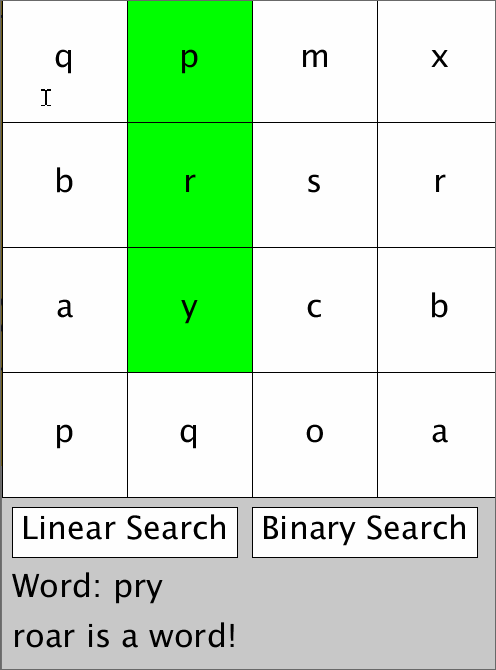
In this game, the user is given a 4x4 block of random characters from which they can spell out words.
2. Requirements
1) Define a class, called Button
, to represent each letter Button
-
Button
contains the following data:-
text (String)
-
x coordinate (float)
-
y coordinate (float)
-
width (float)
-
height (float)
-
isSelected (boolean)
-
-
Button
contains the following methods:-
constructor with parameters for
character
,x
,y
,width
, andheight
.isSelected
should be false initially. -
intersects
method. This method returns true ifmouseX
andmouseY
are inside the bounds of the button and false otherwise -
draw
method. Draws a box button with the text centered in the middle. If the button is selected, draws the box in a different color. -
getSelected
method (Accessor). Returns the value of isSelected. -
setSelected
method (Mutator). Sets the value of isSelected. -
getLabel
method (Accessor). Returns the text on this button.
-
3) In setup, create 16 buttons for the grid of letters as well as buttons for linear and binary search. You are given a function chooseRandomLetter
to help you initialize the values of each letter button. Your base code also loads a dictionary of words to use.
4) In draw, draw your buttons. The base code already draws the helper text.
5) In mousePressed, check whether the user has clicked a button.
-
If they click a character button, append the character to the current word and set the button’s selected property to true.
-
The user should not be allowed to use the same letter block twice!
-
-
If they click a search button, use either linear or binary search to check whether the current word is valid. Then clear the selected buttons and the current word.
6) In binarySearch, implement binary search with String types. (Hint: use the compareTo
function)
7) In linearSearch, implement linear search with String types. (Hint: use the compareTo
function)
3. Starter code
You have basecode for this assignment. Check dropbox.
4. What to hand-in
-
The complete program (Don’t forget your header comment!)
-
A brief write-up with your name, course and assignment number and a few sentences about anything you found challenging about the assignment.
5. Submission guidelines
Submit your program (entire sketch directory), write-up, and image as an electronic copy in the folder marked A05 in your dropbox folder.